Vue2 vs Vue3
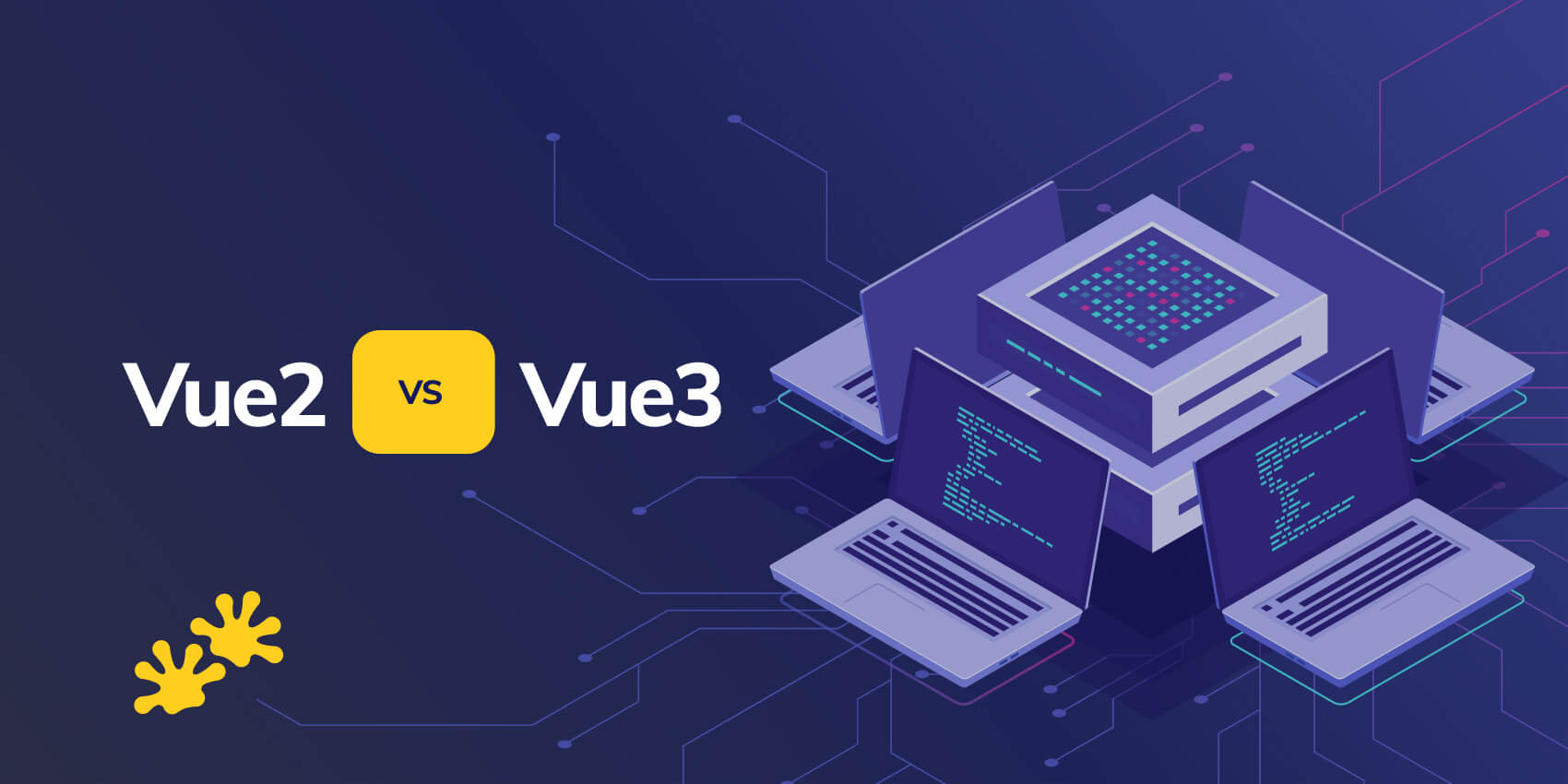
Some of you might hear that the third version of Vue is knocking on the door. Vue.js keeps evolving year by year and Vue 3 is going to be its latest. Vue 3 and Vue 2 go along a similar line, however, there are significant changes in both. The official version of Vue 3 has been released in September 2020. Currently, the APIs, core, structures are stable, and you can use it in production environments.
The question that every Vue.js developer is asking himself is, what are the changes the newest version will bring, and how will they impact the overall performance and stability? Our JS developers took a deeper look at Vue 3, and here are their findings.
Rewrite that makes Vue.js 3 smaller and faster
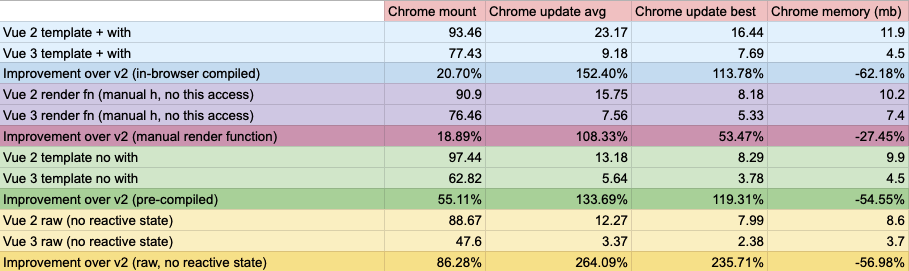
Over the past year, the Vue team has been working on the next major version of Vue.js, which has been released in the second half of 2020. According to the Vue core team, there are two key considerations that led to the new release and rewriting of Vue: first being the general availability of new JavaScript language features in mainstream browsers, and second, being design and architectural issues in the current codebase that had been exposed over time. The rewrite gave the opportunity to rethink the code organization with these things in mind. Upgrades to many aspects of the framework have resulted in speed improvements. Users report that Vue 3 is about 55 percent faster, memory usage is down to 54 percent and updates are up to 133 percent faster. This is achieved by completely rewriting the DOM implementation using TypeScript.
Creating large projects never been easier
A new approach to component logic helps with maintenance and makes writing big projects significantly easier. Vue core team describes Composition API as “a set of additive, function-based APIs that allow flexible composition of component logic“. Vue 3 helps with reducing app complexity, through the years Vue team has noticed that many users are also using Vue to build large scale projects - ones that are iterated on and maintained over a long timeframe, by a team of multiple developers.
Instead of being forced to always organize code by options, code can now be organized as functions, each dealing with a specific feature. The APIs also make it more straightforward to extract and reuse logic between components, or even outside components. It’s also worth noting that full TypeScript support makes large projects maintenance easier than before.
TypeScript
Also, there is a big game-changer, the authors noticed that users were increasingly using Vue and TypeScript together. To support their use cases, they had to author and maintain TypeScript declarations separately from the source code, which used a different type system. Switching to TypeScript allows to automatically generate the declaration files, alleviating the maintenance burden. Vue 3 does not change absolutely with Vue 2 but when completed rewriting it from Vue 2, some expected upgrades turn into a reality and that makes the application smaller, faster, and more powerful. Vue 3’s codebase is written in TypeScript. This enables it to automatically generate, test, and bundle up-to-date type definitions. You can create a Vue app with either TypeScript or JavaScript, depending on your expertise.
interface Book {
title: string
author: string
year: number
}
const Component = defineComponent({
data() {
return {
book: {
title: 'Vue 3 Guide',
author: 'Vue Team',
year: 2020
} as Book
}
}
})
Composition API
So far, there used to be three limitations you may have run into when working with Vue 2:
- As your components get larger readability gets difficult.
- The current code reuse patterns all come with drawbacks.
- Vue 2 has limited TypeScript support out of the box.
The Vue3 Composition API comes with a setup() method. This method encapsulates most of our component code and handles reactivity, lifecycle hooks, and many more. In short, the Composition API helps better organize our code into more modular features rather than separating based on the type of property (methods, computed, data). If you’re familiar with Vue 2, you’ve probably used a mixin for this purpose. But the new Composition API provides a much better solution, readability, and allows Vue applications to be far more scalable.
One of the most interesting parts of the Composition API is that it allows Vue to lean on the safeguards built into native JavaScript in order to share code, like passing variables to functions, and the module system. New API and it’s organization make Vue 3 easier to understand if you were previously working with React, switching to Vue will be easier.
Setup
To use Composition API, we should start with the setup() method.
In Vue 3, you can replace the following options with setup() method:
- Components
- Methods
- Lifecycle methods
- Props
- Data
- Computed Properties
Note: Vue 3 development can use all of the above normally in the same way as Vue 2 without the setup() method.
The new Composition API was rewritten for better logic organization, encapsulations, and reuse which makes it extremely flexible, also tracking the source of every component property is easier.
An example of how a component is structured by using the new API:
<template>
<button @click="increment">
Count is: {{ state.count }}, double is: {{ state.double }}
</button>
</template>
<script>
import { reactive, computed } from 'vue'
export default {
setup() {
const state = reactive({
count: 0,
double: computed(() => state.count * 2)
})
function increment() {
state.count++
}
return {
state,
increment
}
}
}
</script>
The main disadvantage is that it will require some extra time to get familiar with, it’s not as easy as a learning curve that Vue 2 is known for. The good thing is that you don’t need to rewrite your existing components using the new API and you don’t need to use it everywhere as well.
Hooks
Those React components that contain state logic must be a class component. Even if there were already stateless functional components in React there was a need to create components that accommodate state logic without being classes. That’s the moment when the Hooks were born. It uses state logic inside functional components and eliminates the need for writing classes.
Vue.js lets you use functional components that are stateless with mixins, with it, you can define logic or functionality in a particular file and use and even re-use it in a functional component. Vue Hooks are basically an enhanced version of mixins it lets you pass logic from one Hook to another and you can also use state in a Hook from another Hook. Similar to React, Hooks in Vue are defined in functions that can be a cleaner and more flexible way to define and share logic and can return state.
Vue Teleport
Speaking about DOM. Not so common but problematic to solve is having a part of your component mounted in a different position in DOM than the Vue component hierarchy. A scenario for this is for example creating a component that includes a full-screen modal. In most cases, you’d want the modal’s logic to live within the component, but the positioning of the modal quickly becomes difficult to solve through CSS, or requires a change in component composition. This can now easily achieve with the use of the teleport feature like this:
app.component('app-modal', { template: `
<button @click="isOpen = true">
Open modal
</button>
<teleport to="body">
<div v-if="isOpen" class="modal">
I'm a teleported modal
</div>
</teleport>
`,
data() {
return {
isOpen: false
}
}
})
And you can still pass props and interact with it like being inside the component!
Conclusion
Performance has always been and will be essential for frontend frameworks, especially with regard to constantly growing and powerful applications. Now, it’s finally safe to say that Vue 3 is a much more solid framework. It provides a new and simpler way to organize your source code, and with the help of Typescript and their new features for instance Composition API, projects structure can become more readable especially for newcomers. Vue 3 was rewritten, and there are a few long term advantages to this rewrite, though:
The smaller size of the core;
tree-shaking;
optimized slots generation;
hoisting and inlining;
simplification of the source;
it’s easier to learn for newcomers;
better performance - maybe not twice as some say, but still, it’s visible in some scenarios.
There is no chance that we will be able to show you all the changes that Vue 3 brings. The best option is to try it by yourself today.