Vue Composition API vs React Hooks
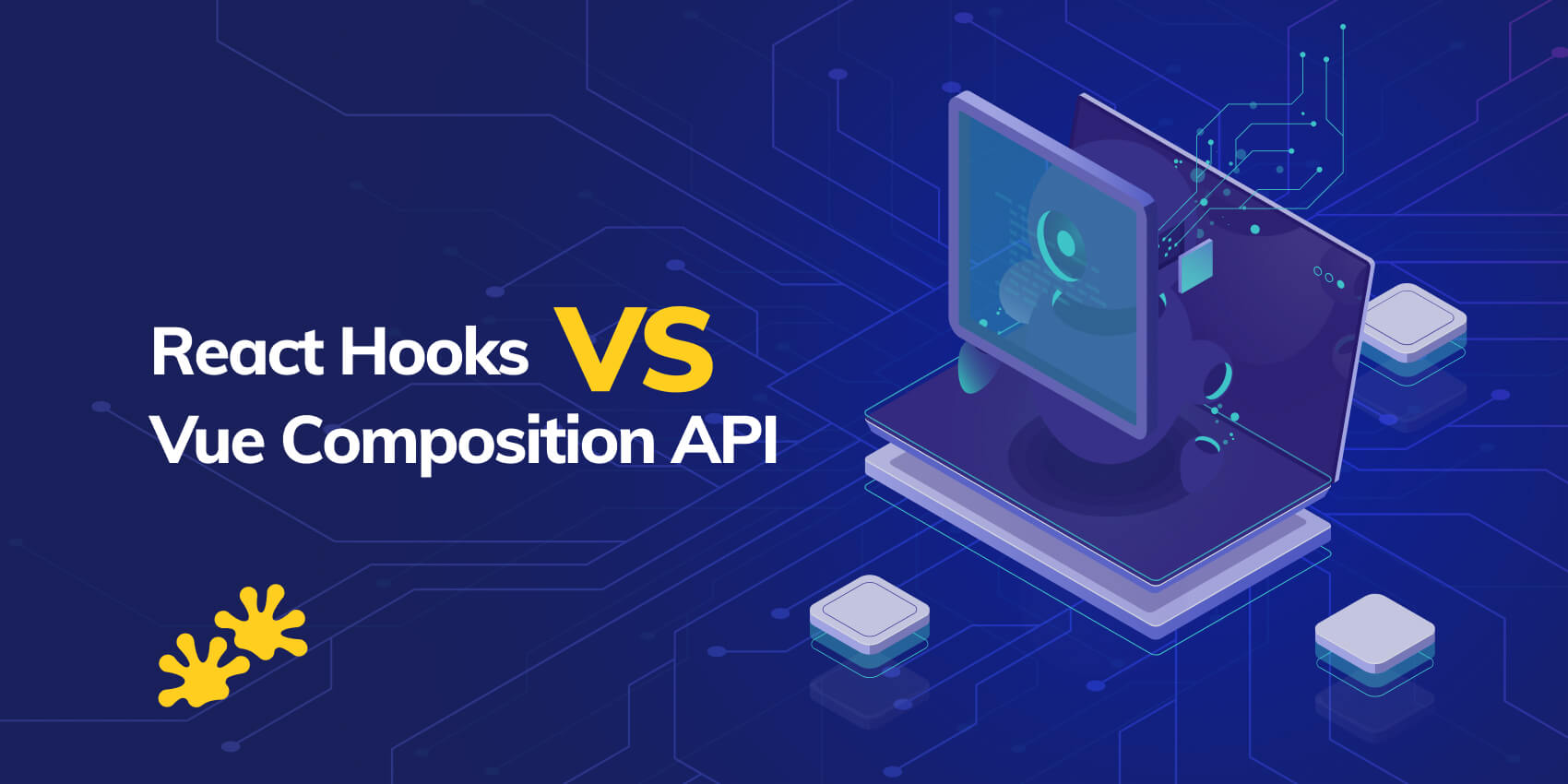
In today’s ever-evolving world of front-end development, Vue Composition API and React Hooks have emerged as powerful tools for building robust and interactive web applications. Both approaches offer developers a more elegant and modular way to manage state and side effects within their codebases. In this blog post, we will dive into the fascinating realm of Vue Composition API and React Hooks, exploring their similarities, differences, and unique features. By comparing these two paradigms, we aim to shed light on their respective strengths and help you make an informed decision on which approach might be the best fit for your next project. So, whether you’re a seasoned Vue enthusiast or a dedicated React aficionado, join us on this journey as we unravel the mysteries of Vue Composition API and React Hooks and unravel the best practices for crafting exceptional web experiences.
React Hooks
React Hooks were introduced at React Conf in October of 2018. Hooks solve a variety of problems in React that have encountered over five years of writing and maintaining tens of thousands of components. It was hard to reuse stateful logic between components, if you had a chance to work with React for a while before introducing Hooks, you may be familiar with patterns like render props and higher-order components that try to solve this. Those techniques required you to restructure your components when you use them, which could be tricky and make code harder to follow.
React Hooks can extract stateful logic from a component, which gives you the possibility to test it independently and reuse it when needed. Hooks allow you to reuse stateful logic without changing your component hierarchy, that makes Hooks easy to use among many components or with the community. At first, the community was rather skeptical, but since then Hooks and Function Components have become the standard way of developing React components for many teams. It also lets you split one component into smaller functions based on what pieces are related, rather than forcing a split based on lifecycle methods.
Vue Composition API
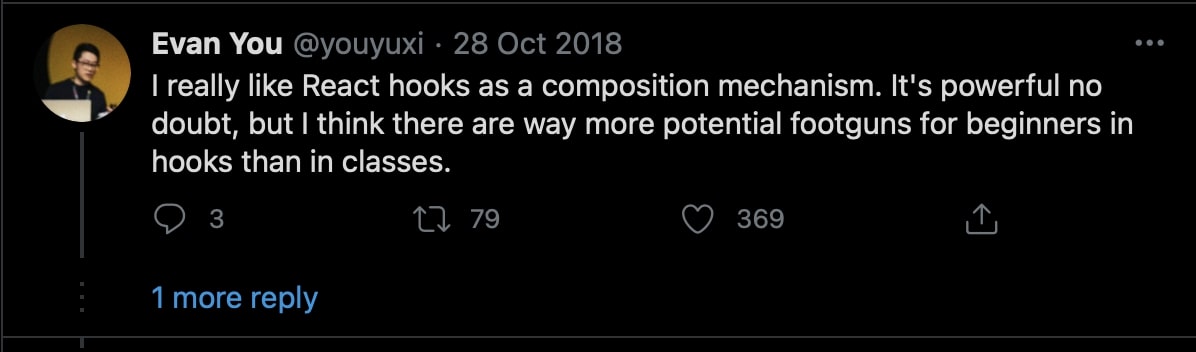
Evan You, the creator of Vue, has described the Composition API as a reactive API coupled with the ability to register lifecycle hooks using globally imported functions. The main thing is that the Composition API provides a different way to manage reactivity at all points in an application. It was introduced in Vue 3, and lets you use functional components that are stateless with mixins, with it you can define logic or functionality in a particular file and use and even re-use it in a functional component. In other words, the Composition API isn't something totally new to the language. You can think about it as exposing some of the internal functions that Vue already uses and allows us to use these functions directly in components.
Hooks in Vue 3 are basically an upgraded version of Mixins. It lets you pass logic from one Hook to another, as well as use state in a Hook from another Hook. Similar to React, Hooks in Vue are defined in functions that result in an easier and more flexible way to define and share logic.
Vue mixins come in handy when you want to feed your component data that needs to be reused in other components. Mixins are flexible in the case of distributing reusable functionalities for Vue.js components. They can contain any component option if it has been used in that component. The idea of mixins works perfectly when working with small-scale applications. As your project gets larger, you may need to create more mixins to handle other kinds of data that will also be reused in other components. That makes a code harder to read and maintain. The Vue Composition API helps solve this issue by enabling you to define whatever function you need in a separate JavaScript file and import it wherever you need to import it to avoid naming conflicts. You can treat Vue Hooks a bit like an upgraded version of Mixins.
Code Readability
Let’s take a look at the differences between coding in React and Vue, as an example, we’ll use Click Counter from React documentation.
That’s the example using React
import { useState } from 'react';
function Example() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked { count} times</p>
<button onClick={ () => setCount(count + 1)} >
Click me
</button>
</div>
);
}
And here is the same example in Vue 3
<script>
import {ref} from ‘vue’
export default {
setup () {
return{
Count: ref(0)
}
}
}
</script>
<template>
<p>You clicked {{count}} times </p>
<button @click=”count += 1”>
Click me
</button>
</template>
As you can notice there are more lines of Vue code, while in the case of React you no longer have individual lines. In general, readability is pretty similar in both examples. The Vue code can be a bit easier to digest. In general, you can say that Vue is not so good in the case of smaller examples like:
// Vue
setup () {
return {
count: ref(0)
}
}
//React
const [count, setCount] = useState(0);
On the other hand, that will change a little bit if you decide to add a few more pieces of state, that’s the moment when the Vue looks like the clearer option.
// Vue
setup () {
return {
count: ref(0),
count2: ref(5),
count3: ref(27)
// React
const [count, setCount] = useState(0);
const [count2, setCount] = useState(5);
const [count3, setCount] = useState(27);
As you can see Vue has a “script” tag and a “template” tag that differentiate them. In React, they save a few lines by putting it all in one function, but it is your role to remember that the setup goes in the main body of the function and the template goes in the return value (except when it is not). It’s hard to decide and almost always it will change a little bit according to actual use of React or Vue. There is one massive difference in case of Vue, check out this fragment.
// Vue
<input type=”text” v-model=”do.it” />
// React
<input
type=”text”
value={do.it}
onChange={e => setName([...do, it: e.target.value)}
/>
While in this example Vue code is pretty clear React has few moving parts that make it less readable. Why is it like that? Well, Vue Team wants to avoid two-way data binding.
The difference in implementation between Vue Composition API and React Hooks
The implementation of Vue Composition API and React Hooks may differ in several aspects, reflecting the unique philosophies and approaches of each framework. Vue Composition API embraces a more declarative and template-centric approach, allowing developers to define their application logic in a highly modular and reusable manner. It promotes the composition of small, self-contained functions called “composition functions“ that encapsulate a specific piece of functionality. These functions can be easily imported and reused across multiple components, enabling code reusability and maintainability. On the other hand, React Hooks follows a more functional programming paradigm, where developers can create custom hooks to encapsulate reusable stateful logic. Hooks are functions that allow developers to “hook into“ React’s lifecycle and state management systems, empowering them to manage state, perform side effects, and compose complex behavior in a more granular manner. React Hooks offer flexibility and fine-grained control over component logic, making it easier to reason about and test individual units of code. In summary, while Vue Composition API emphasizes a template-centric approach with composition functions, React Hooks embrace functional programming principles to provide a more flexible and granular way of managing component logic.
Conclusion
In this article, we explained some of the differences between React Hooks and Composition API. In conclusion, both of these APIs allow developers to create great breathtaking apps. The main difference is how they handle changes of state: While React forces us to keep the data immutable and update it through explicit state setters Vue allows us to simply mutate data by wrapping it into a ref.
It's worth mentioning that it’s an exciting time for both frameworks. Since the introduction of React Hooks in 2018, the community has built amazing things with Custom Hooks on the top. Vue is taking inspiration from React Hooks and adapting them in a little bit different way. We hope you learned something about the approach and philosophies of Vue and React. Both can be used to create astonishing applications, and their differences can be seen as advantages, due to other aims in frameworks philosophy.